npx create-react-app react-test
( 프로젝트 명) 을 통해서 리액트 프로젝트를 생성하면 아래와 같은 구성이 생긴다.
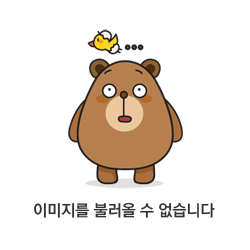
이때 리엑트는 노란색 칠해진 3부분에 의해서 작동한다.
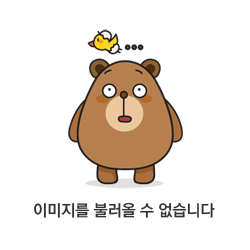
기본적으로 웹페이지는 index.html에서 시작을 하는데 리엑트에서도 마찬가지이다.
하지만 리엑트의 특징은 컴퍼넌트를 통해서 하나의 index.html 페이지에서 컴퍼넌트 교체를 통해서 다른 페이지를 보여주는 거 처럼 작동한다.
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
index.html에 보면 다음과 같이 적혀있다.
이때 npm start(혹은 yarn)대신 직접 열면, root 하위의 파일들이 불러와지지 않아서, 빈 페이지가 보인다.
index.html에 하위 컴퍼넌트를 연결하는 역할을 index.js가 한다.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
이떄 StrictMode는 좀 더 엄격하게 프로젝트를 사용하기 위해 설정하는 것으로, 굳이 없어도 된다.
코드를 보면 html에 있던 id가 root인 곳(body)에 <App/> 을 랜더링한다고 적혀 있다.
그리고 App이 무엇인지는 App.js 파일을 보면 알 수 있다.
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
현재 우리가 봤던 화면은 위 코드를 통해 구성되어있다.
그리고 이를 App.css와 저장된 이미지에 애니메이션 효과를 주어서 우리가 봤던 아래 이미지를 구현한다.
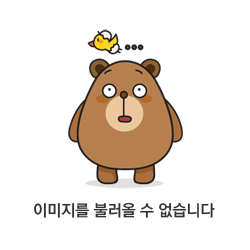
컴퍼넌트는 이때 대문자로 이름이 시작해야하고, export default를 통해서 외부 파일에서 사용할 수 있게 한다.
// index.js의 임포트 부분
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
index.js 에서 ./App 을 통해서 export한 App을 사용하고 있다. 이때 뒤에 './App'; 은 경로인데, .js의 경우는 생략이 가능하다.
'프론트엔드' 카테고리의 다른 글
React swiper 라이브러리 사용 (0) | 2023.08.21 |
---|---|
리엑트 fullCalender 라이브러리 사용 (0) | 2023.08.16 |
리엑트 페이지네이션 라이브러리(react+ SpringBoot) (0) | 2023.08.11 |
form속에 버튼 자동으로 submit되는 거 막기 (0) | 2023.07.24 |
JSP로 게시판 만들기 (0) | 2023.07.02 |